注重体验与质量的电子书资源下载网站
分类于: 设计 计算机基础
简介
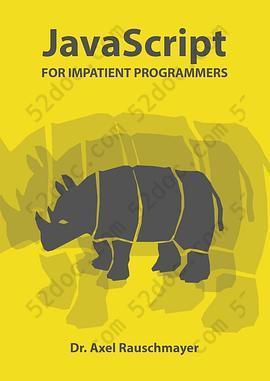
JavaScript for Impatient Programmers 豆 0.0分
资源最后更新于 2020-07-25 14:09:11
作者:Dr. Axel Rauschmayer
出版社:exploringjs.com
出版日期:0000-01
ISBN:9781091210097
文件格式: pdf
标签: JavaScript 计算机 编程 语言 ECMAScript/JavaScript BooksPDF
简介· · · · · ·
This book makes JavaScript less challenging to learn for newcomers, by offering a modern view that is as consistent as possible.
Highlights:
Get started quickly, by initially focusing on modern features.
Test-driven exercises and quizzes available for most chapters.
Covers all essential features of JavaScript, up to and including ES2019.
Optional advanced sections let you dig d...
目录
Table of contents
I. BACKGROUND
1. About this book (ES2019 edition)
1.1. What’s in this book?
1.2. What is not covered by this book?
1.3. About the author
1.4. Acknowledgements
2. FAQ: book
2.1. What should I read if I’m really impatient?
2.2. Why are some chapters and sections marked with “(advanced)”?
2.3. Why are some chapters marked with “(bonus)”?
2.4. How do I submit feedback and corrections?
2.5. How do I get updates for the downloads at Payhip?
2.6. I’m occasionally seeing type annotations – how do those work?
2.7. What do the notes with icons mean?
3. Why JavaScript? (bonus)
3.1. The cons of JavaScript
3.2. The pros of JavaScript
3.3. Pro and con of JavaScript: innovation
4. The nature of JavaScript (bonus)
4.1. JavaScript’s influences
4.2. The nature of JavaScript
4.3. Tips for getting started with JavaScript
5. History and evolution of JavaScript
5.1. How JavaScript was created
5.2. Standardization
5.3. Timeline of ECMAScript versions
5.4. Ecma Technical Committee 39 (TC39)
5.5. The TC39 process
5.6. FAQ: TC39 process
5.7. Evolving JavaScript: don’t break the web
6. FAQ: JavaScript
6.1. What are good references for JavaScript?
6.2. How do I find out what JavaScript features are supported where?
6.3. Where can I look up what features are planned for JavaScript?
6.4. Why does JavaScript fail silently so often?
6.5. Why can’t we clean up JavaScript, by removing quirks and outdated features?
II. FIRST STEPS
7. The big picture
7.1. What are you learning in this book?
7.2. The structure of browsers and Node.js
7.3. Trying out JavaScript code
7.4. JavaScript references
7.5. Further reading
8. Syntax
8.1. An overview of JavaScript’s syntax
8.2. (Advanced)
8.3. Identifiers
8.4. Statement vs. expression
8.5. Ambiguous syntax
8.6. Semicolons
8.7. Automatic semicolon insertion (ASI)
8.8. Semicolons: best practices
8.9. Strict mode
9. Printing information on the console (console.*)
9.1. Printing values: console.log() (stdout)
9.2. Printing error messages: console.error() (stderr)
9.3. Printing nested objects via JSON.stringify()
10. Assertion API
10.1. Assertions in software development
10.2. How assertions are used in this book
10.3. Normal comparison vs. deep comparison
10.4. Quick reference: module assert
11. Getting started with quizzes and exercises
11.1. Quizzes
11.2. Exercises
11.3. Unit tests in JavaScript
III. VARIABLES AND VALUES
12. Variables and assignment
12.1. let
12.2. const
12.3. Deciding between let and const
12.4. The scope of a variable
12.5. (Advanced)
12.6. Terminology: static vs. dynamic
12.7. Temporal dead zone
12.8. Hoisting
12.9. Global variables
12.10. Closures
12.11. Summary: ways of declaring variables
12.12. Further reading
13. Values
13.1. What’s a type?
13.2. JavaScript’s type hierarchy
13.3. The types of the language specification
13.4. Primitive values vs. objects
13.5. Classes and constructor functions
13.6. The operators typeof and instanceof: what’s the type of a value?
13.7. Converting between types
14. Operators
14.1. Two important rules for operators
14.2. The plus operator (+)
14.3. Assignment operators
14.4. Equality: == vs. ===
14.5. Ordering operators
14.6. Various other operators
IV. PRIMITIVE VALUES
15. The non-values undefined and null
15.1. undefined vs. null
15.2. Occurrences of undefined and null
15.3. Checking for undefined or null
15.4. undefined and null don’t have properties
16. Booleans
16.1. Converting to boolean
16.2. Falsy and truthy values
16.3. Conditional operator (? :)
16.4. Binary logical operators: And (x && y), Or (x || y)
16.5. Logical Not (!)
17. Numbers
17.1. JavaScript only has floating point numbers
17.2. Number literals
17.3. Number operators
17.4. Converting to number
17.5. Error values
17.6. Error value: NaN
17.7. Error value: Infinity
17.8. The precision of numbers: careful with decimal fractions
17.9. (Advanced)
17.10. Background: floating point precision
17.11. Integers in JavaScript
17.12. Bitwise operators
17.13. Quick reference: numbers
18. Math
18.1. Data properties
18.2. Exponents, roots, logarithms
18.3. Rounding
18.4. Trigonometric Functions
18.5. asm.js helpers
18.6. Various other functions
18.7. Sources
19. Unicode – a brief introduction (advanced)
19.1. Code points vs. code units
19.2. Web development: UTF-16 and UTF-8
19.3. Grapheme clusters – the real characters
20. Strings
20.1. Plain string literals
20.2. Accessing characters and code points
20.3. String concatenation via +
20.4. Converting to string
20.5. Comparing strings
20.6. Atoms of text: JavaScript characters, code points, grapheme clusters
20.7. Quick reference: Strings
21. Using template literals and tagged templates
21.1. Disambiguation: “template”
21.2. Template literals
21.3. Tagged templates
21.4. Raw string literals
21.5. (Advanced)
21.6. Multi-line template literals and indentation
21.7. Simple templating via template literals
21.8. Further reading
22. Symbols
22.1. Use cases for symbols
22.2. Publicly known symbols
22.3. Converting symbols
22.4. Further reading
V. CONTROL FLOW AND DATA FLOW
23. Control flow statements
23.1. Controlling loops: break and continue
23.2. if statements
23.3. switch statements
23.4. while loops
23.5. do-while loops
23.6. for loops
23.7. for-of loops
23.8. for-await-of loops
23.9. for-in loops (avoid)
24. Exception handling
24.1. Motivation: throwing and catching exceptions
24.2. throw
24.3. try-catch-finally
24.4. Error classes and their properties
25. Callable values
25.1. Kinds of functions
25.2. Ordinary functions
25.3. Specialized functions
25.4. Hoisting functions
25.5. Returning values from functions
25.6. Parameter handling
26. Variable environments (bonus)
26.1. Environment: data structure for managing variables
26.2. Recursion via environments
26.3. Nested scopes via environments
26.4. Closures and environments
VI. MODULARITY
27. Modules
27.1. Before modules: scripts
27.2. Module systems created prior to ES6
27.3. ECMAScript modules
27.4. Named exports
27.5. Default exports
27.6. Naming modules
27.7. Imports are read-only views on exports
27.8. Module specifiers
27.9. Syntactic pitfall: importing is not destructuring
27.10. Preview: loading modules dynamically
27.11. Further reading
28. Single objects
28.1. The two roles of objects in JavaScript
28.2. Objects as records
28.3. Spreading into object literals (...)
28.4. Methods
28.5. Objects as dictionaries
28.6. Standard methods
28.7. Advanced topics
29. Prototype chains and classes
29.1. Prototype chains
29.2. Classes
29.3. Private data for classes
29.4. Subclassing
VII. COLLECTIONS
30. Synchronous iteration
30.1. What is synchronous iteration about?
30.2. Core iteration constructs: iterables and iterators
30.3. Iterating manually
30.4. Iteration in practice
30.5. Quick reference: synchronous iteration
30.6. Further reading
31. Arrays (Array)
31.1. The two roles of Arrays in JavaScript
31.2. Basic Array operations
31.3. for-of and Arrays
31.4. Array-like objects
31.5. Converting iterable and Array-like values to Arrays
31.6. Creating and filling Arrays with arbitrary lengths
31.7. Multidimensional Arrays
31.8. More Array features (advanced)
31.9. Adding and removing elements (destructively and non-destructively)
31.10. Methods: iteration and transformation (.find(), .map(), .filter(), etc.)
31.11. .sort(): sorting Arrays
31.12. Quick reference: Array<T>
32. Typed Arrays: handling binary data (Advanced)
32.1. The basics of the API
32.2. Foundations of the Typed Array API
32.3. ArrayBuffers
32.4. Typed Arrays
32.5. DataViews
32.6. Further reading
33. Maps (Map)
33.1. Using Maps
33.2. Example: Counting characters
33.3. A few more details about the keys of Maps (advanced)
33.4. Missing Map operations
33.5. Quick reference: Map<K,V>
33.6. FAQ
34. WeakMaps (WeakMap)
34.1. Attaching values to objects via WeakMaps
34.2. WeakMaps as black boxes
34.3. Examples
34.4. WeakMap API
35. Sets (Set)
35.1. Using Sets
35.2. Comparing Set elements
35.3. Missing Set operations
35.4. Quick reference: Set<T>
36. WeakSets (WeakSet)
36.1. Example: Marking objects as safe to use with a method
36.2. WeakSet API
37. Destructuring
37.1. A first taste of destructuring
37.2. Constructing vs. extracting
37.3. Where can we destructure?
37.4. Object-destructuring
37.5. Array-destructuring
37.6. Destructuring use case: multiple return values
37.7. Not finding a match
37.8. What values can’t be destructured?
37.9. (Advanced)
37.10. Default values
37.11. Parameter definitions are similar to destructuring
37.12. Nested destructuring
37.13. Further reading
38. Synchronous generators (advanced)
38.1. What are synchronous generators?
38.2. Calling generators from generators (advanced)
38.3. Example: Reusing loops
38.4. Advanced features of generators
VIII. ASYNCHRONICITY
39. Asynchronous programming in JavaScript
39.1. A roadmap for asynchronous programming in JavaScript
39.2. The call stack
39.3. The event loop
39.4. How to avoid blocking the JavaScript process
39.5. Patterns for delivering asynchronous results
39.6. Asynchronous code: the downsides
39.7. Resources
40. Promises for asynchronous programming
40.1. The basics of using Promises
40.2. Examples
40.3. Error handling: don’t mix rejections and exceptions
40.4. Promise-based functions start synchronously, settle asynchronously
40.5. Promise.all(): concurrency and Arrays of Promises
40.6. Tips for chaining Promises
40.7. Further reading
41. Async functions
41.1. Async functions: the basics
41.2. Terminology
41.3. await is shallow (you can’t use it in callbacks)
41.4. (Advanced)
41.5. Immediately invoked async arrow functions
41.6. Concurrency and await
41.7. Tips for using async functions
42. Asynchronous iteration
42.1. Basic asynchronous iteration
42.2. Asynchronous generators
42.3. Async iteration over Node.js streams
IX. MORE STANDARD LIBRARY
43. Regular expressions (RegExp)
43.1. Creating regular expressions
43.2. Syntax
43.3. Flags
43.4. Properties of regular expression objects
43.5. Methods for working with regular expressions
43.6. Flag /g and its pitfalls
43.7. Techniques for working with regular expressions
44. Dates (Date)
44.1. Best practice: don’t use the current built-in API
44.2. Background: UTC vs. GMT
44.3. Background: date time formats (ISO)
44.4. Time values
44.5. Creating Dates
44.6. Getters and setters
44.7. Converting Dates to strings
44.8. Pitfalls of the Date API
45. Creating and parsing JSON (JSON)
45.1. The discovery and standardization of JSON
45.2. JSON syntax
45.3. Using the JSON API
45.4. Configuring what is stringified or parsed (advanced)
45.5. FAQ
45.6. Quick reference: JSON
X. MISCELLANEOUS TOPICS
46. Next steps: overview of web development (bonus)
46.1. Tips against feeling overwhelmed
46.2. Things worth learning for web development
46.3. Example: a tool-based JavaScript workflow
46.4. An overview of JavaScript tools
46.5. Tools not related to JavaScript
46.6. Further reading on JavaScript
XI. APPENDICES
A. Index
I. BACKGROUND
1. About this book (ES2019 edition)
1.1. What’s in this book?
1.2. What is not covered by this book?
1.3. About the author
1.4. Acknowledgements
2. FAQ: book
2.1. What should I read if I’m really impatient?
2.2. Why are some chapters and sections marked with “(advanced)”?
2.3. Why are some chapters marked with “(bonus)”?
2.4. How do I submit feedback and corrections?
2.5. How do I get updates for the downloads at Payhip?
2.6. I’m occasionally seeing type annotations – how do those work?
2.7. What do the notes with icons mean?
3. Why JavaScript? (bonus)
3.1. The cons of JavaScript
3.2. The pros of JavaScript
3.3. Pro and con of JavaScript: innovation
4. The nature of JavaScript (bonus)
4.1. JavaScript’s influences
4.2. The nature of JavaScript
4.3. Tips for getting started with JavaScript
5. History and evolution of JavaScript
5.1. How JavaScript was created
5.2. Standardization
5.3. Timeline of ECMAScript versions
5.4. Ecma Technical Committee 39 (TC39)
5.5. The TC39 process
5.6. FAQ: TC39 process
5.7. Evolving JavaScript: don’t break the web
6. FAQ: JavaScript
6.1. What are good references for JavaScript?
6.2. How do I find out what JavaScript features are supported where?
6.3. Where can I look up what features are planned for JavaScript?
6.4. Why does JavaScript fail silently so often?
6.5. Why can’t we clean up JavaScript, by removing quirks and outdated features?
II. FIRST STEPS
7. The big picture
7.1. What are you learning in this book?
7.2. The structure of browsers and Node.js
7.3. Trying out JavaScript code
7.4. JavaScript references
7.5. Further reading
8. Syntax
8.1. An overview of JavaScript’s syntax
8.2. (Advanced)
8.3. Identifiers
8.4. Statement vs. expression
8.5. Ambiguous syntax
8.6. Semicolons
8.7. Automatic semicolon insertion (ASI)
8.8. Semicolons: best practices
8.9. Strict mode
9. Printing information on the console (console.*)
9.1. Printing values: console.log() (stdout)
9.2. Printing error messages: console.error() (stderr)
9.3. Printing nested objects via JSON.stringify()
10. Assertion API
10.1. Assertions in software development
10.2. How assertions are used in this book
10.3. Normal comparison vs. deep comparison
10.4. Quick reference: module assert
11. Getting started with quizzes and exercises
11.1. Quizzes
11.2. Exercises
11.3. Unit tests in JavaScript
III. VARIABLES AND VALUES
12. Variables and assignment
12.1. let
12.2. const
12.3. Deciding between let and const
12.4. The scope of a variable
12.5. (Advanced)
12.6. Terminology: static vs. dynamic
12.7. Temporal dead zone
12.8. Hoisting
12.9. Global variables
12.10. Closures
12.11. Summary: ways of declaring variables
12.12. Further reading
13. Values
13.1. What’s a type?
13.2. JavaScript’s type hierarchy
13.3. The types of the language specification
13.4. Primitive values vs. objects
13.5. Classes and constructor functions
13.6. The operators typeof and instanceof: what’s the type of a value?
13.7. Converting between types
14. Operators
14.1. Two important rules for operators
14.2. The plus operator (+)
14.3. Assignment operators
14.4. Equality: == vs. ===
14.5. Ordering operators
14.6. Various other operators
IV. PRIMITIVE VALUES
15. The non-values undefined and null
15.1. undefined vs. null
15.2. Occurrences of undefined and null
15.3. Checking for undefined or null
15.4. undefined and null don’t have properties
16. Booleans
16.1. Converting to boolean
16.2. Falsy and truthy values
16.3. Conditional operator (? :)
16.4. Binary logical operators: And (x && y), Or (x || y)
16.5. Logical Not (!)
17. Numbers
17.1. JavaScript only has floating point numbers
17.2. Number literals
17.3. Number operators
17.4. Converting to number
17.5. Error values
17.6. Error value: NaN
17.7. Error value: Infinity
17.8. The precision of numbers: careful with decimal fractions
17.9. (Advanced)
17.10. Background: floating point precision
17.11. Integers in JavaScript
17.12. Bitwise operators
17.13. Quick reference: numbers
18. Math
18.1. Data properties
18.2. Exponents, roots, logarithms
18.3. Rounding
18.4. Trigonometric Functions
18.5. asm.js helpers
18.6. Various other functions
18.7. Sources
19. Unicode – a brief introduction (advanced)
19.1. Code points vs. code units
19.2. Web development: UTF-16 and UTF-8
19.3. Grapheme clusters – the real characters
20. Strings
20.1. Plain string literals
20.2. Accessing characters and code points
20.3. String concatenation via +
20.4. Converting to string
20.5. Comparing strings
20.6. Atoms of text: JavaScript characters, code points, grapheme clusters
20.7. Quick reference: Strings
21. Using template literals and tagged templates
21.1. Disambiguation: “template”
21.2. Template literals
21.3. Tagged templates
21.4. Raw string literals
21.5. (Advanced)
21.6. Multi-line template literals and indentation
21.7. Simple templating via template literals
21.8. Further reading
22. Symbols
22.1. Use cases for symbols
22.2. Publicly known symbols
22.3. Converting symbols
22.4. Further reading
V. CONTROL FLOW AND DATA FLOW
23. Control flow statements
23.1. Controlling loops: break and continue
23.2. if statements
23.3. switch statements
23.4. while loops
23.5. do-while loops
23.6. for loops
23.7. for-of loops
23.8. for-await-of loops
23.9. for-in loops (avoid)
24. Exception handling
24.1. Motivation: throwing and catching exceptions
24.2. throw
24.3. try-catch-finally
24.4. Error classes and their properties
25. Callable values
25.1. Kinds of functions
25.2. Ordinary functions
25.3. Specialized functions
25.4. Hoisting functions
25.5. Returning values from functions
25.6. Parameter handling
26. Variable environments (bonus)
26.1. Environment: data structure for managing variables
26.2. Recursion via environments
26.3. Nested scopes via environments
26.4. Closures and environments
VI. MODULARITY
27. Modules
27.1. Before modules: scripts
27.2. Module systems created prior to ES6
27.3. ECMAScript modules
27.4. Named exports
27.5. Default exports
27.6. Naming modules
27.7. Imports are read-only views on exports
27.8. Module specifiers
27.9. Syntactic pitfall: importing is not destructuring
27.10. Preview: loading modules dynamically
27.11. Further reading
28. Single objects
28.1. The two roles of objects in JavaScript
28.2. Objects as records
28.3. Spreading into object literals (...)
28.4. Methods
28.5. Objects as dictionaries
28.6. Standard methods
28.7. Advanced topics
29. Prototype chains and classes
29.1. Prototype chains
29.2. Classes
29.3. Private data for classes
29.4. Subclassing
VII. COLLECTIONS
30. Synchronous iteration
30.1. What is synchronous iteration about?
30.2. Core iteration constructs: iterables and iterators
30.3. Iterating manually
30.4. Iteration in practice
30.5. Quick reference: synchronous iteration
30.6. Further reading
31. Arrays (Array)
31.1. The two roles of Arrays in JavaScript
31.2. Basic Array operations
31.3. for-of and Arrays
31.4. Array-like objects
31.5. Converting iterable and Array-like values to Arrays
31.6. Creating and filling Arrays with arbitrary lengths
31.7. Multidimensional Arrays
31.8. More Array features (advanced)
31.9. Adding and removing elements (destructively and non-destructively)
31.10. Methods: iteration and transformation (.find(), .map(), .filter(), etc.)
31.11. .sort(): sorting Arrays
31.12. Quick reference: Array<T>
32. Typed Arrays: handling binary data (Advanced)
32.1. The basics of the API
32.2. Foundations of the Typed Array API
32.3. ArrayBuffers
32.4. Typed Arrays
32.5. DataViews
32.6. Further reading
33. Maps (Map)
33.1. Using Maps
33.2. Example: Counting characters
33.3. A few more details about the keys of Maps (advanced)
33.4. Missing Map operations
33.5. Quick reference: Map<K,V>
33.6. FAQ
34. WeakMaps (WeakMap)
34.1. Attaching values to objects via WeakMaps
34.2. WeakMaps as black boxes
34.3. Examples
34.4. WeakMap API
35. Sets (Set)
35.1. Using Sets
35.2. Comparing Set elements
35.3. Missing Set operations
35.4. Quick reference: Set<T>
36. WeakSets (WeakSet)
36.1. Example: Marking objects as safe to use with a method
36.2. WeakSet API
37. Destructuring
37.1. A first taste of destructuring
37.2. Constructing vs. extracting
37.3. Where can we destructure?
37.4. Object-destructuring
37.5. Array-destructuring
37.6. Destructuring use case: multiple return values
37.7. Not finding a match
37.8. What values can’t be destructured?
37.9. (Advanced)
37.10. Default values
37.11. Parameter definitions are similar to destructuring
37.12. Nested destructuring
37.13. Further reading
38. Synchronous generators (advanced)
38.1. What are synchronous generators?
38.2. Calling generators from generators (advanced)
38.3. Example: Reusing loops
38.4. Advanced features of generators
VIII. ASYNCHRONICITY
39. Asynchronous programming in JavaScript
39.1. A roadmap for asynchronous programming in JavaScript
39.2. The call stack
39.3. The event loop
39.4. How to avoid blocking the JavaScript process
39.5. Patterns for delivering asynchronous results
39.6. Asynchronous code: the downsides
39.7. Resources
40. Promises for asynchronous programming
40.1. The basics of using Promises
40.2. Examples
40.3. Error handling: don’t mix rejections and exceptions
40.4. Promise-based functions start synchronously, settle asynchronously
40.5. Promise.all(): concurrency and Arrays of Promises
40.6. Tips for chaining Promises
40.7. Further reading
41. Async functions
41.1. Async functions: the basics
41.2. Terminology
41.3. await is shallow (you can’t use it in callbacks)
41.4. (Advanced)
41.5. Immediately invoked async arrow functions
41.6. Concurrency and await
41.7. Tips for using async functions
42. Asynchronous iteration
42.1. Basic asynchronous iteration
42.2. Asynchronous generators
42.3. Async iteration over Node.js streams
IX. MORE STANDARD LIBRARY
43. Regular expressions (RegExp)
43.1. Creating regular expressions
43.2. Syntax
43.3. Flags
43.4. Properties of regular expression objects
43.5. Methods for working with regular expressions
43.6. Flag /g and its pitfalls
43.7. Techniques for working with regular expressions
44. Dates (Date)
44.1. Best practice: don’t use the current built-in API
44.2. Background: UTC vs. GMT
44.3. Background: date time formats (ISO)
44.4. Time values
44.5. Creating Dates
44.6. Getters and setters
44.7. Converting Dates to strings
44.8. Pitfalls of the Date API
45. Creating and parsing JSON (JSON)
45.1. The discovery and standardization of JSON
45.2. JSON syntax
45.3. Using the JSON API
45.4. Configuring what is stringified or parsed (advanced)
45.5. FAQ
45.6. Quick reference: JSON
X. MISCELLANEOUS TOPICS
46. Next steps: overview of web development (bonus)
46.1. Tips against feeling overwhelmed
46.2. Things worth learning for web development
46.3. Example: a tool-based JavaScript workflow
46.4. An overview of JavaScript tools
46.5. Tools not related to JavaScript
46.6. Further reading on JavaScript
XI. APPENDICES
A. Index