注重体验与质量的电子书资源下载网站
分类于: 设计 编程语言
简介
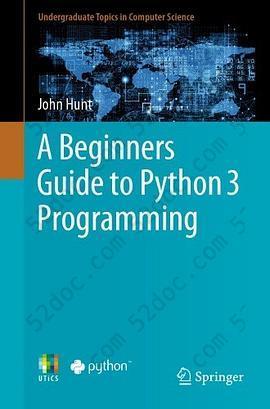
A Beginners Guide To Python 3 Programming: Undergraduate Topics In Computer Science 豆 0.0分
资源最后更新于 2020-07-25 14:09:36
作者:John Hunt
出版社:Springer
出版日期:0000-01
ISBN:9783030202897
文件格式: pdf
简介· · · · · ·
This textbook on Python 3 explains concepts such as variables and what they represent, how data is held in memory, how a for loop works and what a string is. It also introduces key concepts such as functions, modules and packages as well as object orientation and functional programming. Each section is prefaced with an introductory chapter, before continuing with how these idea...
目录
Preface......Page 7
What You Need......Page 8
Downloading the PyCharm IDE......Page 9
Setting Up the IDE......Page 10
Conventions......Page 12
Example Code and Sample Solutions......Page 13
Contents......Page 14
1.1 What Is Python?......Page 27
1.2 Python Versions......Page 28
1.4 Python Libraries......Page 29
1.5 Python Execution Model......Page 30
1.6.1 Interactively Using the Python Interpreter......Page 31
1.6.2 Running a Python File......Page 32
1.6.3 Executing a Python Script......Page 33
1.6.4 Using Python in an IDE......Page 35
1.7 Useful Resources......Page 36
2.2 Check to See If Python Is Installed......Page 38
2.3 Installing Python on a Windows PC......Page 40
2.4 Setting Up on a Mac......Page 45
2.5 Online Resources......Page 47
3.2 Hello World......Page 48
3.3 Interactive Hello World......Page 49
3.4 Variables......Page 51
3.5 Naming Conventions......Page 53
3.7 Python Statements......Page 54
3.9 Scripts Versus Programs......Page 55
3.11 Exercises......Page 56
4.2 What Are Strings?......Page 57
4.3 Representing Strings......Page 58
4.5 What Can You Do with Strings?......Page 59
4.5.3 Accessing a Character......Page 60
4.5.5 Repeating Strings......Page 61
4.5.7 Counting Strings......Page 62
4.5.9 Finding Sub Strings......Page 63
4.5.12 Other String Operations......Page 64
4.6.3 Function/Method Invocations......Page 66
4.7 String Formatting......Page 67
4.8 String Templates......Page 69
4.9 Online Resources......Page 72
4.10 Exercises......Page 73
5.2 Types of Numbers......Page 74
5.3 Integers......Page 75
5.4 Floating Point Numbers......Page 76
5.4.2 Converting an Input String into a Floating Point Number......Page 77
5.6 Boolean Values......Page 78
5.7.1 Integer Operations......Page 80
5.7.2 Negative Number Integer Division......Page 82
5.7.4 Integers and Floating Point Operations......Page 83
5.8 Assignment Operators......Page 84
5.9 None Value......Page 85
5.11 Exercises......Page 86
5.11.2 Convert Kilometres to Miles......Page 87
6.2 Comparison Operators......Page 88
6.4 The If Statement......Page 89
6.4.1 Working with an If Statement......Page 90
6.4.3 The Use of elif......Page 91
6.5 Nesting If Statements......Page 92
6.6 If Expressions......Page 93
6.7 A Note on True and False......Page 94
6.10.1 Check Input Is Positive or Negative......Page 95
6.10.3 Kilometres to Miles Converter......Page 96
7.2 While Loop......Page 97
7.3 For Loop......Page 99
7.4 Break Loop Statement......Page 101
7.5 Continue Loop Statement......Page 103
7.6 For Loop with Else......Page 104
7.8 Dice Roll Game......Page 105
7.10.1 Calculate the Factorial of a Number......Page 106
7.10.2 Print All the Prime Numbers in a Range......Page 107
8.2.1 Add a Welcome Message......Page 108
8.2.2 Running the Program......Page 109
8.3 What Will the Program Do?......Page 110
8.4.1 Generate the Random Number......Page 111
8.4.3 Check to See If the Player Has Guessed the Number......Page 112
8.4.4 Check They Haven’t Exceeded Their Maximum Number of Guess......Page 113
8.4.5 Notify the Player Whether Higher or Lower......Page 114
8.5 The Complete Listing......Page 115
8.6.2 Blank Lines Within a Block of Code......Page 117
8.7 Exercises......Page 118
9.2 Recursive Behaviour......Page 119
9.4 Recursively Searching a Tree......Page 120
9.6 Calculating Factorial Recursively......Page 122
9.7 Disadvantages of Recursion......Page 124
9.9 Exercises......Page 125
10.2 Structured Analysis and Function Identification......Page 127
10.3 Functional Decomposition......Page 128
10.3.1 Functional Decomposition Terminology......Page 129
10.3.3 Calculator Functional Decomposition Example......Page 130
10.5 Data Flow Diagrams......Page 132
10.6 Flowcharts......Page 133
10.7 Data Dictionary......Page 135
10.8 Online Resources......Page 136
11.2 What Are Functions?......Page 137
11.4 Types of Functions......Page 138
11.5 Defining Functions......Page 139
11.5.1 An Example Function......Page 140
11.6 Returning Values from Functions......Page 141
11.7 Docstring......Page 143
11.8.1 Multiple Parameter Functions......Page 144
11.8.2 Default Parameter Values......Page 145
11.8.3 Named Arguments......Page 146
11.8.4 Arbitrary Arguments......Page 147
11.8.5 Positional and Keyword Arguments......Page 148
11.9 Anonymous Functions......Page 149
11.11 Exercises......Page 151
12.2 Local Variables......Page 152
12.3 The Global Keyword......Page 154
12.4 Nonlocal Variables......Page 155
12.5 Hints......Page 156
12.7 Exercise......Page 157
13.2 What the Calculator Will Do......Page 158
13.4 The Calculator Operations......Page 159
13.5 Behaviour of the Calculator......Page 160
13.6 Identifying Whether the User Has Finished......Page 161
13.7 Selecting the Operation......Page 163
13.8 Obtaining the Input Numbers......Page 165
13.10 Running the Calculator......Page 166
13.11 Exercises......Page 167
14.2 What Is Functional Programming?......Page 168
14.3 Advantages to Functional Programming......Page 170
14.5 Referential Transparency......Page 172
14.6 Further Reading......Page 174
15.2 Recap on Functions in Python......Page 175
15.3 Functions as Objects......Page 176
15.4 Higher Order Function Concepts......Page 178
15.4.1 Higher Order Function Example......Page 179
15.5 Python Higher Order Functions......Page 180
15.5.1 Using Higher Order Functions......Page 181
15.5.2 Functions Returning Functions......Page 182
15.7 Exercises......Page 183
16.2 Currying Concepts......Page 185
16.3 Python and Curried Functions......Page 186
16.4 Closures......Page 188
16.5 Online Resources......Page 190
16.6 Exercises......Page 191
17.2 Classes......Page 192
17.3 What Are Classes for?......Page 193
17.3.2 Class Terminology......Page 194
17.4 How Is an OO System Constructed?......Page 195
17.4.1 Where Do We Start?......Page 196
17.4.2 Identifying the Objects......Page 197
17.4.3 Identifying the Services or Methods......Page 198
17.4.4 Refining the Objects......Page 199
17.4.5 Bringing It All Together......Page 200
17.5 Where Is the Structure in an OO Program?......Page 203
17.6 Further Reading......Page 205
18.1 Introduction......Page 206
18.2 Class Definitions......Page 207
18.3 Creating Examples of the Class Person......Page 208
18.4 Be Careful with Assignment......Page 209
18.5.1 Accessing Object Attributes......Page 211
18.5.2 Defining a Default String Representation......Page 212
18.6 Providing a Class Comment......Page 213
18.7 Adding a Birthday Method......Page 214
18.8 Defining Instance Methods......Page 215
18.9 Person Class Recap......Page 216
18.10 The del Keyword......Page 217
18.11 Automatic Memory Management......Page 218
18.12 Intrinsic Attributes......Page 219
18.14 Exercises......Page 220
19.2 Class Side Data......Page 222
19.3 Class Side Methods......Page 223
19.4 Static Methods......Page 224
19.6 Online Resources......Page 225
19.7 Exercises......Page 226
20.2 What Is Inheritance?......Page 227
20.3 Terminology Around Inheritance......Page 231
20.4 The Class Object and Inheritance......Page 233
20.6 Purpose of Subclasses......Page 234
20.7 Overriding Methods......Page 235
20.8 Extending Superclass Methods......Page 237
20.9 Inheritance Oriented Naming Conventions......Page 238
20.10 Python and Multiple Inheritance......Page 239
20.11 Multiple Inheritance Considered Harmful......Page 241
20.13 Online Resources......Page 246
20.14 Exercises......Page 247
21.2 The Procedural Approach......Page 249
21.2.1 Procedures for the Data Structure......Page 250
21.3.1 Packages Versus Classes......Page 251
21.3.2 Inheritance......Page 253
21.4 Summary......Page 255
22.2.1 Why Have Operator Overloading?......Page 256
22.2.3 Implementing Operator Overloading......Page 257
22.3 Numerical Operators......Page 259
22.4 Comparison Operators......Page 262
22.6 Summary......Page 264
22.8 Exercises......Page 265
23.2 Python Attributes......Page 267
23.3 Setter and Getter Style Methods......Page 269
23.4 Public Interface to Properties......Page 270
23.5 More Concise Property Definitions......Page 272
23.7 Exercises......Page 274
24.2 Errors and Exceptions......Page 276
24.3 What Is an Exception?......Page 277
24.4 What Is Exception Handling?......Page 278
24.5 Handling an Exception......Page 280
24.5.1 Accessing the Exception Object......Page 282
24.5.2 Jumping to Exception Handlers......Page 283
24.5.4 The Else Clause......Page 285
24.5.5 The Finally Clause......Page 286
24.6 Raising an Exception......Page 287
24.7 Defining an Custom Exception......Page 288
24.8 Chaining Exceptions......Page 290
24.10 Exercises......Page 292
25.2 Modules......Page 294
25.3 Python Modules......Page 295
25.4.1 Importing a Module......Page 297
25.4.2 Importing from a Module......Page 298
25.4.3 Hiding Some Elements of a Module......Page 300
25.5 Module Properties......Page 301
25.6 Standard Modules......Page 302
25.7 Python Module Search Path......Page 304
25.8 Modules as Scripts......Page 305
25.9.1 Package Organisation......Page 307
25.10 Online Resources......Page 309
25.11 Exercise......Page 310
26.2 Abstract Classes as a Concept......Page 311
26.3.1 Subclassing an ABC......Page 312
26.3.2 Defining an Abstract Base Class......Page 314
26.4 Defining an Interface......Page 316
26.5 Virtual Subclasses......Page 317
26.6 Mixins......Page 318
26.8 Exercises......Page 320
27.2 Implicit Contracts......Page 322
27.3 Duck Typing......Page 324
27.4 Protocols......Page 325
27.6 The Context Manager Protocol......Page 326
27.7 Polymorphism......Page 328
27.8 The Descriptor Protocol......Page 330
27.10 Exercises......Page 333
28.2 What Is Monkey Patching?......Page 335
28.2.2 Monkey Patching Example......Page 336
28.2.3 The Self Parameter......Page 337
28.3 Attribute Lookup......Page 338
28.4 Handling Unknown Attribute Access......Page 341
28.5 Handling Unknown Method Invocations......Page 342
28.6 Intercepting Attribute Lookup......Page 343
28.7 Intercepting Setting an Attribute......Page 344
28.9 Exercises......Page 345
29.2 What Are Decorators?......Page 347
29.3 Defining a Decorator......Page 348
29.4 Using Decorators......Page 349
29.6 Stacked Decorators......Page 350
29.7 Parameterised Decorators......Page 352
29.8.1 Methods Without Parameters......Page 353
29.8.2 Methods with Parameters......Page 354
29.9 Class Decorators......Page 355
29.10 When Is a Decorator Executed?......Page 357
29.12 FuncTools Wrap......Page 358
29.13 Online Resources......Page 359
29.15 Exercises......Page 360
30.2.1 Iterables......Page 362
30.2.4 The Iterable Evens Class......Page 363
30.2.5 Using the Evens Class with a for Loop......Page 364
30.4.1 Defining a Generator Function......Page 365
30.4.3 When Do the Yield Statements Execute?......Page 366
30.4.5 Nesting Generator Functions......Page 367
30.4.6 Using Generators Outside a for Loop......Page 368
30.5 Coroutines......Page 369
30.7 Exercises......Page 370
31.2 Python Collection Types......Page 372
31.3.2 The tuple() Constructor Function......Page 373
31.3.4 Creating New Tuples from Existing Tuples......Page 374
31.3.5 Tuples Can Hold Different Types......Page 375
31.3.7 Tuple Related Functions......Page 376
31.3.9 Nested Tuples......Page 377
31.4.1 Creating Lists......Page 378
31.4.2 List Constructor Function......Page 380
31.4.3 Accessing Elements from a List......Page 381
31.4.4 Adding to a List......Page 382
31.4.6 List Concatenation......Page 383
31.4.8 The pop() Method......Page 384
31.4.9 Deleting from a List......Page 385
31.5 Online Resources......Page 386
31.6 Exercises......Page 387
32.2 Creating a Set......Page 388
32.5.1 Checking for Presence of an Element......Page 389
32.5.3 Changing Items in a Set......Page 390
32.5.6 Removing an Item......Page 391
32.5.7 Nesting Sets......Page 392
32.6 Set Operations......Page 393
32.8 Online Resources......Page 395
32.9 Exercises......Page 396
33.2 Creating a Dictionary......Page 397
33.2.1 The dict() Constructor Function......Page 398
33.3.3 Changing a Keys Value......Page 399
33.3.4 Removing an Entry......Page 400
33.3.5 Iterating over Keys......Page 401
33.3.7 Checking Key Membership......Page 402
33.3.9 Nesting Dictionaries......Page 403
33.4 A Note on Dictionary Key Objects......Page 404
33.6 Online Resources......Page 405
33.7 Exercises......Page 406
34.2 List Comprehension......Page 409
34.3 The Collections Module......Page 410
34.4 The Itertools Module......Page 413
34.6 Exercises......Page 414
35.2 Abstract Data Types......Page 415
35.4 Queues......Page 416
35.4.2 Defining a Queue Class......Page 417
35.5 Stacks......Page 419
35.5.1 Python List as a Stack......Page 420
35.7 Exercises......Page 421
36.2 Filter......Page 423
36.3 Map......Page 425
36.4 Reduce......Page 427
36.6 Exercises......Page 428
37.2 Classes in the Game......Page 430
37.3 Counter Class......Page 433
37.5 The Player Class......Page 434
37.6 The HumanPlayer Class......Page 435
37.7 The ComputerPlayer Class......Page 436
37.8 The Board Class......Page 437
37.9 The Game Class......Page 438
37.10 Running the Game......Page 439
What You Need......Page 8
Downloading the PyCharm IDE......Page 9
Setting Up the IDE......Page 10
Conventions......Page 12
Example Code and Sample Solutions......Page 13
Contents......Page 14
1.1 What Is Python?......Page 27
1.2 Python Versions......Page 28
1.4 Python Libraries......Page 29
1.5 Python Execution Model......Page 30
1.6.1 Interactively Using the Python Interpreter......Page 31
1.6.2 Running a Python File......Page 32
1.6.3 Executing a Python Script......Page 33
1.6.4 Using Python in an IDE......Page 35
1.7 Useful Resources......Page 36
2.2 Check to See If Python Is Installed......Page 38
2.3 Installing Python on a Windows PC......Page 40
2.4 Setting Up on a Mac......Page 45
2.5 Online Resources......Page 47
3.2 Hello World......Page 48
3.3 Interactive Hello World......Page 49
3.4 Variables......Page 51
3.5 Naming Conventions......Page 53
3.7 Python Statements......Page 54
3.9 Scripts Versus Programs......Page 55
3.11 Exercises......Page 56
4.2 What Are Strings?......Page 57
4.3 Representing Strings......Page 58
4.5 What Can You Do with Strings?......Page 59
4.5.3 Accessing a Character......Page 60
4.5.5 Repeating Strings......Page 61
4.5.7 Counting Strings......Page 62
4.5.9 Finding Sub Strings......Page 63
4.5.12 Other String Operations......Page 64
4.6.3 Function/Method Invocations......Page 66
4.7 String Formatting......Page 67
4.8 String Templates......Page 69
4.9 Online Resources......Page 72
4.10 Exercises......Page 73
5.2 Types of Numbers......Page 74
5.3 Integers......Page 75
5.4 Floating Point Numbers......Page 76
5.4.2 Converting an Input String into a Floating Point Number......Page 77
5.6 Boolean Values......Page 78
5.7.1 Integer Operations......Page 80
5.7.2 Negative Number Integer Division......Page 82
5.7.4 Integers and Floating Point Operations......Page 83
5.8 Assignment Operators......Page 84
5.9 None Value......Page 85
5.11 Exercises......Page 86
5.11.2 Convert Kilometres to Miles......Page 87
6.2 Comparison Operators......Page 88
6.4 The If Statement......Page 89
6.4.1 Working with an If Statement......Page 90
6.4.3 The Use of elif......Page 91
6.5 Nesting If Statements......Page 92
6.6 If Expressions......Page 93
6.7 A Note on True and False......Page 94
6.10.1 Check Input Is Positive or Negative......Page 95
6.10.3 Kilometres to Miles Converter......Page 96
7.2 While Loop......Page 97
7.3 For Loop......Page 99
7.4 Break Loop Statement......Page 101
7.5 Continue Loop Statement......Page 103
7.6 For Loop with Else......Page 104
7.8 Dice Roll Game......Page 105
7.10.1 Calculate the Factorial of a Number......Page 106
7.10.2 Print All the Prime Numbers in a Range......Page 107
8.2.1 Add a Welcome Message......Page 108
8.2.2 Running the Program......Page 109
8.3 What Will the Program Do?......Page 110
8.4.1 Generate the Random Number......Page 111
8.4.3 Check to See If the Player Has Guessed the Number......Page 112
8.4.4 Check They Haven’t Exceeded Their Maximum Number of Guess......Page 113
8.4.5 Notify the Player Whether Higher or Lower......Page 114
8.5 The Complete Listing......Page 115
8.6.2 Blank Lines Within a Block of Code......Page 117
8.7 Exercises......Page 118
9.2 Recursive Behaviour......Page 119
9.4 Recursively Searching a Tree......Page 120
9.6 Calculating Factorial Recursively......Page 122
9.7 Disadvantages of Recursion......Page 124
9.9 Exercises......Page 125
10.2 Structured Analysis and Function Identification......Page 127
10.3 Functional Decomposition......Page 128
10.3.1 Functional Decomposition Terminology......Page 129
10.3.3 Calculator Functional Decomposition Example......Page 130
10.5 Data Flow Diagrams......Page 132
10.6 Flowcharts......Page 133
10.7 Data Dictionary......Page 135
10.8 Online Resources......Page 136
11.2 What Are Functions?......Page 137
11.4 Types of Functions......Page 138
11.5 Defining Functions......Page 139
11.5.1 An Example Function......Page 140
11.6 Returning Values from Functions......Page 141
11.7 Docstring......Page 143
11.8.1 Multiple Parameter Functions......Page 144
11.8.2 Default Parameter Values......Page 145
11.8.3 Named Arguments......Page 146
11.8.4 Arbitrary Arguments......Page 147
11.8.5 Positional and Keyword Arguments......Page 148
11.9 Anonymous Functions......Page 149
11.11 Exercises......Page 151
12.2 Local Variables......Page 152
12.3 The Global Keyword......Page 154
12.4 Nonlocal Variables......Page 155
12.5 Hints......Page 156
12.7 Exercise......Page 157
13.2 What the Calculator Will Do......Page 158
13.4 The Calculator Operations......Page 159
13.5 Behaviour of the Calculator......Page 160
13.6 Identifying Whether the User Has Finished......Page 161
13.7 Selecting the Operation......Page 163
13.8 Obtaining the Input Numbers......Page 165
13.10 Running the Calculator......Page 166
13.11 Exercises......Page 167
14.2 What Is Functional Programming?......Page 168
14.3 Advantages to Functional Programming......Page 170
14.5 Referential Transparency......Page 172
14.6 Further Reading......Page 174
15.2 Recap on Functions in Python......Page 175
15.3 Functions as Objects......Page 176
15.4 Higher Order Function Concepts......Page 178
15.4.1 Higher Order Function Example......Page 179
15.5 Python Higher Order Functions......Page 180
15.5.1 Using Higher Order Functions......Page 181
15.5.2 Functions Returning Functions......Page 182
15.7 Exercises......Page 183
16.2 Currying Concepts......Page 185
16.3 Python and Curried Functions......Page 186
16.4 Closures......Page 188
16.5 Online Resources......Page 190
16.6 Exercises......Page 191
17.2 Classes......Page 192
17.3 What Are Classes for?......Page 193
17.3.2 Class Terminology......Page 194
17.4 How Is an OO System Constructed?......Page 195
17.4.1 Where Do We Start?......Page 196
17.4.2 Identifying the Objects......Page 197
17.4.3 Identifying the Services or Methods......Page 198
17.4.4 Refining the Objects......Page 199
17.4.5 Bringing It All Together......Page 200
17.5 Where Is the Structure in an OO Program?......Page 203
17.6 Further Reading......Page 205
18.1 Introduction......Page 206
18.2 Class Definitions......Page 207
18.3 Creating Examples of the Class Person......Page 208
18.4 Be Careful with Assignment......Page 209
18.5.1 Accessing Object Attributes......Page 211
18.5.2 Defining a Default String Representation......Page 212
18.6 Providing a Class Comment......Page 213
18.7 Adding a Birthday Method......Page 214
18.8 Defining Instance Methods......Page 215
18.9 Person Class Recap......Page 216
18.10 The del Keyword......Page 217
18.11 Automatic Memory Management......Page 218
18.12 Intrinsic Attributes......Page 219
18.14 Exercises......Page 220
19.2 Class Side Data......Page 222
19.3 Class Side Methods......Page 223
19.4 Static Methods......Page 224
19.6 Online Resources......Page 225
19.7 Exercises......Page 226
20.2 What Is Inheritance?......Page 227
20.3 Terminology Around Inheritance......Page 231
20.4 The Class Object and Inheritance......Page 233
20.6 Purpose of Subclasses......Page 234
20.7 Overriding Methods......Page 235
20.8 Extending Superclass Methods......Page 237
20.9 Inheritance Oriented Naming Conventions......Page 238
20.10 Python and Multiple Inheritance......Page 239
20.11 Multiple Inheritance Considered Harmful......Page 241
20.13 Online Resources......Page 246
20.14 Exercises......Page 247
21.2 The Procedural Approach......Page 249
21.2.1 Procedures for the Data Structure......Page 250
21.3.1 Packages Versus Classes......Page 251
21.3.2 Inheritance......Page 253
21.4 Summary......Page 255
22.2.1 Why Have Operator Overloading?......Page 256
22.2.3 Implementing Operator Overloading......Page 257
22.3 Numerical Operators......Page 259
22.4 Comparison Operators......Page 262
22.6 Summary......Page 264
22.8 Exercises......Page 265
23.2 Python Attributes......Page 267
23.3 Setter and Getter Style Methods......Page 269
23.4 Public Interface to Properties......Page 270
23.5 More Concise Property Definitions......Page 272
23.7 Exercises......Page 274
24.2 Errors and Exceptions......Page 276
24.3 What Is an Exception?......Page 277
24.4 What Is Exception Handling?......Page 278
24.5 Handling an Exception......Page 280
24.5.1 Accessing the Exception Object......Page 282
24.5.2 Jumping to Exception Handlers......Page 283
24.5.4 The Else Clause......Page 285
24.5.5 The Finally Clause......Page 286
24.6 Raising an Exception......Page 287
24.7 Defining an Custom Exception......Page 288
24.8 Chaining Exceptions......Page 290
24.10 Exercises......Page 292
25.2 Modules......Page 294
25.3 Python Modules......Page 295
25.4.1 Importing a Module......Page 297
25.4.2 Importing from a Module......Page 298
25.4.3 Hiding Some Elements of a Module......Page 300
25.5 Module Properties......Page 301
25.6 Standard Modules......Page 302
25.7 Python Module Search Path......Page 304
25.8 Modules as Scripts......Page 305
25.9.1 Package Organisation......Page 307
25.10 Online Resources......Page 309
25.11 Exercise......Page 310
26.2 Abstract Classes as a Concept......Page 311
26.3.1 Subclassing an ABC......Page 312
26.3.2 Defining an Abstract Base Class......Page 314
26.4 Defining an Interface......Page 316
26.5 Virtual Subclasses......Page 317
26.6 Mixins......Page 318
26.8 Exercises......Page 320
27.2 Implicit Contracts......Page 322
27.3 Duck Typing......Page 324
27.4 Protocols......Page 325
27.6 The Context Manager Protocol......Page 326
27.7 Polymorphism......Page 328
27.8 The Descriptor Protocol......Page 330
27.10 Exercises......Page 333
28.2 What Is Monkey Patching?......Page 335
28.2.2 Monkey Patching Example......Page 336
28.2.3 The Self Parameter......Page 337
28.3 Attribute Lookup......Page 338
28.4 Handling Unknown Attribute Access......Page 341
28.5 Handling Unknown Method Invocations......Page 342
28.6 Intercepting Attribute Lookup......Page 343
28.7 Intercepting Setting an Attribute......Page 344
28.9 Exercises......Page 345
29.2 What Are Decorators?......Page 347
29.3 Defining a Decorator......Page 348
29.4 Using Decorators......Page 349
29.6 Stacked Decorators......Page 350
29.7 Parameterised Decorators......Page 352
29.8.1 Methods Without Parameters......Page 353
29.8.2 Methods with Parameters......Page 354
29.9 Class Decorators......Page 355
29.10 When Is a Decorator Executed?......Page 357
29.12 FuncTools Wrap......Page 358
29.13 Online Resources......Page 359
29.15 Exercises......Page 360
30.2.1 Iterables......Page 362
30.2.4 The Iterable Evens Class......Page 363
30.2.5 Using the Evens Class with a for Loop......Page 364
30.4.1 Defining a Generator Function......Page 365
30.4.3 When Do the Yield Statements Execute?......Page 366
30.4.5 Nesting Generator Functions......Page 367
30.4.6 Using Generators Outside a for Loop......Page 368
30.5 Coroutines......Page 369
30.7 Exercises......Page 370
31.2 Python Collection Types......Page 372
31.3.2 The tuple() Constructor Function......Page 373
31.3.4 Creating New Tuples from Existing Tuples......Page 374
31.3.5 Tuples Can Hold Different Types......Page 375
31.3.7 Tuple Related Functions......Page 376
31.3.9 Nested Tuples......Page 377
31.4.1 Creating Lists......Page 378
31.4.2 List Constructor Function......Page 380
31.4.3 Accessing Elements from a List......Page 381
31.4.4 Adding to a List......Page 382
31.4.6 List Concatenation......Page 383
31.4.8 The pop() Method......Page 384
31.4.9 Deleting from a List......Page 385
31.5 Online Resources......Page 386
31.6 Exercises......Page 387
32.2 Creating a Set......Page 388
32.5.1 Checking for Presence of an Element......Page 389
32.5.3 Changing Items in a Set......Page 390
32.5.6 Removing an Item......Page 391
32.5.7 Nesting Sets......Page 392
32.6 Set Operations......Page 393
32.8 Online Resources......Page 395
32.9 Exercises......Page 396
33.2 Creating a Dictionary......Page 397
33.2.1 The dict() Constructor Function......Page 398
33.3.3 Changing a Keys Value......Page 399
33.3.4 Removing an Entry......Page 400
33.3.5 Iterating over Keys......Page 401
33.3.7 Checking Key Membership......Page 402
33.3.9 Nesting Dictionaries......Page 403
33.4 A Note on Dictionary Key Objects......Page 404
33.6 Online Resources......Page 405
33.7 Exercises......Page 406
34.2 List Comprehension......Page 409
34.3 The Collections Module......Page 410
34.4 The Itertools Module......Page 413
34.6 Exercises......Page 414
35.2 Abstract Data Types......Page 415
35.4 Queues......Page 416
35.4.2 Defining a Queue Class......Page 417
35.5 Stacks......Page 419
35.5.1 Python List as a Stack......Page 420
35.7 Exercises......Page 421
36.2 Filter......Page 423
36.3 Map......Page 425
36.4 Reduce......Page 427
36.6 Exercises......Page 428
37.2 Classes in the Game......Page 430
37.3 Counter Class......Page 433
37.5 The Player Class......Page 434
37.6 The HumanPlayer Class......Page 435
37.7 The ComputerPlayer Class......Page 436
37.8 The Board Class......Page 437
37.9 The Game Class......Page 438
37.10 Running the Game......Page 439